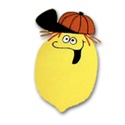
So I just got out of a meeting with my manager's manager. My manager is trying to rebuild an existing system - as opposed to simply augmenting it, and the way he's going about doing this is by using AWS services and a flock of 11 consultants. They are skipping what is, and jumping right to what he wants, and in so doing, leaving all the employees of the company that might offer help, support, and certainly ongoing maintenance - completely in the dark.
I've asked for details about the project I'm supposed to be on, and the response was always "None of your concern." OK... you're the manager. After repeated attempts to talk to HR, and seeing nothing done about it, I've simply taken the stand that I'll be very up-front about the fact that I'm doing nothing, as long as I'm given nothing to do. That way people can choose to see that as an anomaly - or not. Their choice.
I'm being paid either way. I'm very transparent with HR so that should it come back to me that I was wasting company resources (my time), that would be documented as not true. So I'm covered.
I don't think that using AWS is wrong. It's just a different approach than what I know the CTO has expressly stated: It just costs too much. It's more, but if you factor in the cost of having a datacenter, and racks, and machines, and people to maintain them, and then people to install, upgrade, monitor and fix the services like AWS offers, well... I'm not certain that all cases are clearly cheaper - one way or the other. I really think that the costs are coming down, and the service levels are to the point that you need to consider it on a case-by-case basis to be 100% certain.
But that's just my opinion, and not the CTO's. And he has made himself very clear on the matter. So for my manager (a consultant) to come in and make the plan, and hire the consultants to execute this plan that is 100% AWS... well... I knew it'd be a point of contention, and told my manager this when he first showed me his plans.
"Not your concern. That's something I'll make happen."
OK, boss... I tried to tell you. As my GrandPa Bowen said:
Those who don't listen, must feel.
-- Don Bowen
so he's going to have to feel it.
Part of that seems to be happening in the meeting I just got out of. The CTO said that AWS was not going to happen. That the enhancement of the existing system was the job, and that I was going to be brought in - as well as the other employees that have a solid understanding of the existing system. Makes good sense to me.
But it's 180 degrees from what my manager has been doing, planning, and moving towards.
So starting tomorrow I can see a new round in the fight. There's no way that the current plan is going to be allowed. So what value are the AWS consultants? My manager is being forced to bring in people he didn't want to bring in - and actively excluded and rejected. How hard are we going to work for this guy? Why should we? He was ready to drop us all - and told me as much less than a week ago. So how is he going to make this happen?
At the same time, the first round went to him in hiring the consultants, and starting this work... but this last round went to the CTO who shut down a $2 million deployment because we already had 90% of it in-house, paid for, and supported. It would be a colossal waste of money.
I'm no fool... this fight isn't over, just a temporary lull in the action. Soon there will be more slings and arrows, and we that are caught in the middle will no doubt soon tire of this, and let the (non-)Titans battle it out amongst themselves.
But is this any way to run a company?