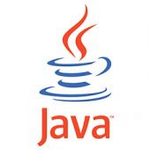
Well... today has been a very eventful day. I had noticed that Oracle dropped support for JDK 10, and mentioned it to some friends in Slack. I didn't know the details, so I decided to dig in and see if I could see anything on the net about it. I did. Lots. Changed my day.
Turns out, Oracle is changing it's licensing for JDK 11 - it's free for development and testing, but if you use it in production, it requires a license. A paid license. Yikes!
OpenJDK is also built by Oracle, and IBM, and RedHat - and it is Open Source - free for any use-case. So that's where people appear to be going. I can see that, but I've stayed away from OpenJDK because it's just the official JDK from Oracle. But now it seems Oracle is looking to cash in on the JDK, and this is just a lovely predicament.
But there is hope - kinda. Homebrew is supporting OpenJDK as a cask so that it can be versioned, and supported within the Homebrew ecosystem. That's good news. To install the latest, you simply need to:
$ brew cask install java
and it will install it into:
$ brew cask info java
java: 11.0.2,9
https://jdk.java.net/
/usr/local/Caskroom/java/11.0.2,9 (64B)
From: https://github.com/Homebrew/homebrew-cask/blob/master/Casks/java.rb
==> Name
OpenJDK Java Development Kit
==> Artifacts
jdk-11.0.2.jdk -> /Library/Java/JavaVirtualMachines/openjdk-11.0.2.jdk
(Generic Artifact)
and the tools I built for switching JDK versions work just fine:
$ setjdk 1.8; echo $JAVA_HOME
/Library/Java/JavaVirtualMachines/jdk1.8.0_181.jdk/Contents/Home
$ setjdk 11; echo $JAVA_HOME
/Library/Java/JavaVirtualMachines/openjdk-11.0.2.jdk/Contents/Home
That's the good news. What isn't really good is that there is an issue with the Java Collections where the toArray method added an additional arity and that breaks Clojure - badly. So it really doesn't help to have the OpenJDK 11 on the box - as Clojure can't make use of it.
However, that's not all... it appears that when I upgraded Leiningen, I broke one of the really nice plugins that I like to use with it - Ultra. The error is nasty as well:
$ lein clean
$ lein deps
$ lein repl
[WARNING] No nREPL middleware descriptor in metadata of
#'clojure.tools.nrepl.middleware.render-values/render-values,
see nrepl.middleware/set-descriptor!
[WARNING] No nREPL middleware descriptor in metadata of
#'clojure.tools.nrepl.middleware.render-values/render-values,
see nrepl.middleware/set-descriptor!
nREPL server started on port 54967 on host 127.0.0.1 - nrepl://127.0.0.1:54967
ERROR: Unhandled REPL handler exception processing message
{:id ad3092dd-8491-4d60-a1a9-5092d4d090a9, :op clone}
java.lang.IllegalArgumentException: No implementation of method:
:send of protocol: #'nrepl.transport/Transport
and if I removed Ultra from my ~/.lein/profiles.clj then everything worked fine again. But this was with Leiningen 2.9.0 - and that was an issue. In fact, it was an issue that's already been reported to Ultra. I added my notes, as the suggestion didn't work for me.
So now I'm left with downgrading Leiningen to get Clojure working again. This is not as easy as I'd hoped, but it's what needs to be done. First, get into where the Homebrew formulae are stored, :
$ cd $(brew --prefix)/Homebrew/Library/Taps/homebrew/homebrew-core/Formula
$ git log --follow leiningen.rb
commit 2be19f3787d811247095e704765fc33a8815d639
Author: BrewTestBot <homebrew-test-bot@lists.sfconservancy.org>
Date: Tue Feb 12 13:07:29 2019 +0000
leiningen: update 2.9.0 bottle.
commit 9ca7899818eb02cc8252d47cc32b5a42554a7655
Author: Rahul De <rahul080327@gmail.com>
Date: Tue Feb 12 10:43:59 2019 +0100
leiningen 2.9.0
Closes #36927.
Signed-off-by: Chongyu Zhu <i@lembacon.com>
commit fd1c0ba8f162a3c41debef6143dca8ba19227f90
Author: BrewTestBot <homebrew-test-bot@lists.sfconservancy.org>
Date: Sat Dec 15 10:45:41 2018 +0000
leiningen: update 2.8.3 bottle.
commit 40553be0b01710db9e16cff978f593b83ecdfe3b
Author: Rahul De <rahul080327@gmail.com>
Date: Sat Dec 15 11:27:08 2018 +0100
Leiningen 2.8.3
Closes #35151.
at this point we heed the git SHA for the version we want to move to. In the case of Leiningen, the SHA for 2.8.3 is fd1c0ba8f162a3c41debef6143dca8ba19227f90 and the SHA for 2.7.1 is cdddd1094b26d0092e0030051dac97a286bb3fc4. I started with 2.8.3, and that didn't work, so I had to go back to 2.7.1. This is a big difference.
Then make a branch for that version, and reinstall Leininden from the Ruby file:
$ git checkout -b leiningen-2.8.3 fd1c0ba8
$ brew reinstall ./leiningen.rb
$ brew switch leiningen 2.8.3
$ git checkout master
for 2.8.3 and, for 2.7.1:
$ git checkout -b leiningen-2.7.1 cdddd109
$ brew reinstall ./leiningen.rb
$ brew switch leiningen 2.7.1
$ git checkout master
At this point, we can see that both versions are installed:
$ brew info leiningen
leiningen: stable 2.7.1 (bottled), HEAD
Build tool for Clojure
https://github.com/technomancy/leiningen
/usr/local/Cellar/leiningen/2.7.1 (9 files, 14.7MB) *
Poured from bottle on 2019-02-18 at 13:29:34
/usr/local/Cellar/leiningen/2.8.3 (9 files, 13MB)
Poured from bottle on 2019-02-18 at 13:22:14
Now it's possible to run Leiningen and Ultra - again. It has to be JDK 8, and Leiningen 2.7.1... but until Ultra is fixed for the change in Leiningen, this is as far as I can go.
What was even more annoying was that my new work laptop has a very recent version of Homebrew on it, and the git repo has been trimmed, and so there is no git SHAs for the previous versions. However, with tar and copying the tarball over, I was able to get the 2.7.1 and 2.8.3 versions there, and things are now working OK.
Wow! What a mess to get back to where I thought I started the day!