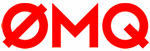
The design we have for my ticker plant is to have the different products and exchanges spread out over a large number of reliable multicast channels, address and port combinations. In order to do this with ZeroMQ, I was under the impression that I needed to have a zmq::socket_t for each channel - which in ZeroMQ terms is really a URL like: epgm://eth0;225.1.1.1:55555. After all, the basic code for a multicast receiver looks like:
#include <zmq.hpp>
// make a ZeroMQ context to handle all this - use one thread for I/O
zmq::context_t context(1);
// make a simple socket, and connect it to the multicast channel
zmq::socket_t socket(context, ZMQ_SUB);
socket.connect("epgm://eth0;225.1.1.1:55555");
// now set it up for all subscriptions
socket.setsockopt(ZMQ_SUBSCRIBE, "", 0);
// receive a single message
zmq::message_t msg;
socket.recv(&msg);
So in many ways, the ZeroMQ socket looks and acts like a regular socket. But I've read in many of the mailing list posts, and even talking to a guy here at The Shop that has dug into the code, that these aren't really sockets - they are just the logical way the ZeroMQ guys made their code appear to the users of their stuff.
This is never more obvious than the mailing list post I read today about having multiple connections to a single zmq::socket_t. The maintainer of the library said that there can be multiple connections to a single socket:
#include <zmq.hpp>
// make a ZeroMQ context to handle all this - use one thread for I/O
zmq::context_t context(1);
// make a simple socket, and connect it to the multicast channel
zmq::socket_t socket(context, ZMQ_SUB);
socket.connect("epgm://eth0;225.1.1.1:55555");
socket.connect("epgm://eth0;225.1.1.1:55666");
socket.connect("epgm://eth0;225.1.1.1:77777");
socket.connect("epgm://eth0;225.1.1.1:88888");
// now set it up for all subscriptions
socket.setsockopt(ZMQ_SUBSCRIBE, "", 0);
// receive a single message
zmq::message_t msg;
socket.recv(&msg);
and the recv() will then pick off the first available message on any one of the connected sockets. That's great news! As opposed to needing n sockets for n channels, I can have just one, and that simplifies my code a huge amount.
So that's what I'm working on. I'm nearly done, and then I can test. Can't wait to see how this works.