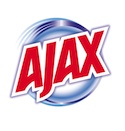
I got a request for my web app to add in user-based permissioning. Basically, the management wanted to restrict the data any one person in the Shop might see based on their login name. Since I'd already done the user authentication with a signed Java applet, it seemed like a natural extension to add in the portfolios that the individual user could see. I simply change the JavaScript code to parse the response and then went back to the java servlet and added in the database lookup of a new column of data from the database table with the list of usernames.
I decided that it was easiest, for now, to just have a semi-colon-separated list of values, the first would be the YES or NO of the approval, and if YES, then the remainder would be the names of the allowed portfolios for this user. This was taken straight out of the database column.
Like I said, simple.
As I get more requests, I'll probably try to make it JSON and have that allow for the map-like capabilities of the basic JavaScript object. At that time, I'll move to returning JavaScript and then running eval(xhr.responseTest) in the return method. That will make it easier to extend in the future. The only wrinkle is that I'll have to assume that there's a standard variable that the response is coded to, but that's not horrible.
When I got the servlet working, and the simple parsing of the results, I then set about to allow the user to see only that portion of the data that was contained in the portfolio list I'd just parsed. This, as it turns out, was pretty easy.
The first thing I did was to get rid of the repetition in the HTML and JavaScript for all the individual portfolios. There were check boxes, checks on the data, etc. All those needed to be automated and made expandable simply. When I looked at the code, it was pretty simple. Leave all the checkboxes as-is, and create a simple JavaScript array of the names of the portfolios. Then, in the initialChecks() call, use document.getElementById() where the id was the name of the portfolio. Simple.
The tougher part was making the checkboxes disappear when the user shouldn't be able to see their data. The answer was really rather simple: make a div that surrounds the checkbox and name it "div_Portfolio". That way I can make an array of them and get their references by again using document.getElementById('div_' + portfolio). Then, I can make them invisible by:
// get all the references of the checkboxes and enclosing divs
var portfolioChecks = [];
var portfolioDivs = [];
for (var i = 0; i < portfolioNames.length; ++i) {
portfolioChecks[i] = document.getElementById(portfolioNames[i]);
portfolioDivs[i] = document.getElementById('div_' + portfolioNames[i]);
}
// ...get the list of the available portfolios
// remove the portfolios that the user can't see
for (var i = 0; i < portfolioNames.length; ++i) {
if (response.indexOf(portfolioNames[i]) < 0) {
portfolioChecks[i].checked = false;
portfolioDivs[i].style.display = 'none';
}
}
with this 'turn off, then remove' code, I was able to leave the large part of the app unchanged and it just worked. I was very pleased with the way it turned out.
Now I have something that looks good for all users, and they can see only the data the management wants them to see. Lovely.